How To Import Module with Module Name Str in Python
GOAL
To import modules by its name as below.
def import_n_th_module(index): """ the function to get index number and import the module named mymodule+index such as mymodule1, mymodule2,... """ module_name = 'mymodule' + str(index) # import module_name => the error occured
Environment
Python 3.8.7
Method
Use importlib package.
The purpose of the importlib package is two-fold. One is to provide the implementation of the import statement (and thus, by extension, the import() function) in Python source code. <omit>
from importlib — The implementation of import of Python documentaion
You can import module with importlib.import_module() as follows.
importlib.import_module('numpy') # output => import numpy module
Example
I created 3 modules in modules package. And each module prints “[module_name] is imported” when being imported.
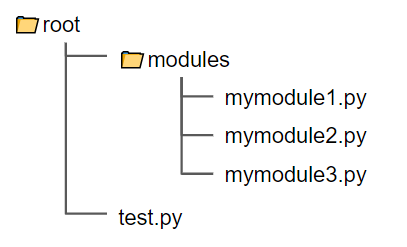
#test.py import sys import importlib index = 3 importlib.import_module('modules.mymodule' + str(index)) # output => "mymodule3 is imported"
Postscript
Another purpose of the imporlib
Two, the components to implement import are exposed in this package, making it easier for users to create their own custom objects (known generically as an importer) to participate in the import process.
from importlib — The implementation of import of Python documentaion
You can see all methods of importlib in importlib — The implementation of import of Python documentaion.