How to fit the widget to the parent layout in PySide2
GOAL
To fit the sizes of the children widgets to the layout of the parent. In other words, to remove the space around widgets from the layout.
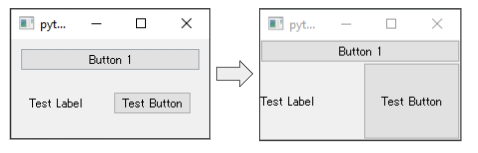
Environment
Windows 10
Python 3.8.7
PySide2 5.15.2
Method
There are 2 steps to delete margin space from the layout.
- Set the margin of the layout 0
- Set the spacing of the layout 0
Create widget and set layout
I created a sample widget as follows.
import sys from PySide2.QtWidgets import * class MyMainWindow(QMainWindow): def __init__(self, parent=None): super(MyMainWindow, self).__init__(parent) self._generateUI() def _generateUI(self): main_widget = QWidget() main_layout = QVBoxLayout() main_widget.setLayout(main_layout) self.setCentralWidget(main_widget) button1 = QPushButton("Button 1") main_layout.addWidget(button1) sub_widget = QWidget() sub_layout = QHBoxLayout() sub_widget.setLayout(sub_layout) label = QLabel("Test Label") button = QPushButton("Test Button") button.setSizePolicy(QSizePolicy.Minimum, QSizePolicy.Expanding) sub_layout.addWidget(label) sub_layout.addWidget(button) main_layout.addWidget(sub_widget) def launch(): app = QApplication.instance() if not app: app = QApplication(sys.argv) widget = MyMainWindow() widget.show() app.exec_() launch()
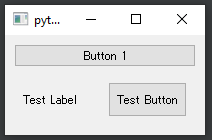
1. Set the margin of the layout 0
Set the margin 0 with setMargin() function.
main_layout.setMargin(0) sub_layout.setMargin(0)
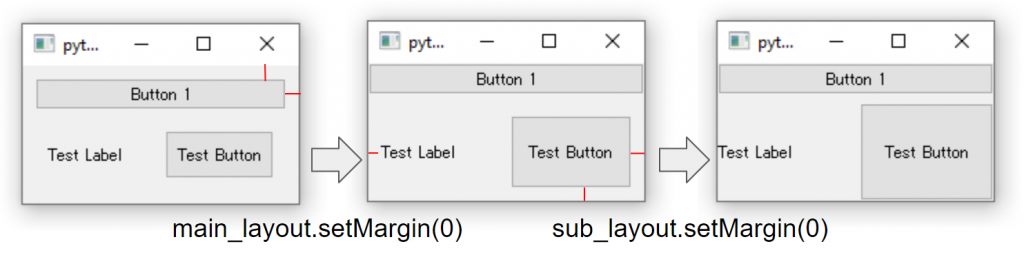
2. Set the spacing of the layout 0
Set the spacing 0 with setSpacing() function. Spacing is the space between item widget in the BoxLayout.
main_layout.setSpacing(0) sub_layout.setSpacing(0)
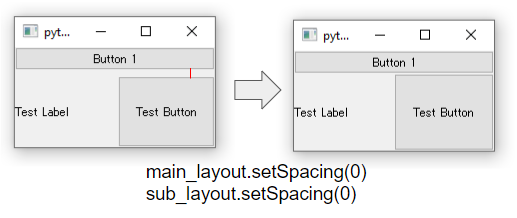