What is gettext
Gettext is an library for internationalization(I18N) and localization(L10N).
GOAL
To understand what is gettext and how to use it.
What is gettext
gettext is a library for translation in software. It can make sentences in the software multilingual.
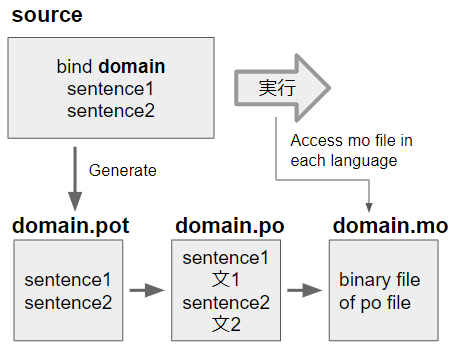
pot file
Pot file is the template file for translation. Sentences to translate are defined in it.
po file
Translations of the sentences defined in pot file in each language are listed in po file.
mo file
mo file is binary file generated from po file.
domain
Domain is the identifier with that gettext module find the template for traslation.
How to use gettext in Python
Use gettext module.
Method 1. GNU gettext API
This method can work only in linux and unix because it uses the environment variables LANGUAGE, LC_ALL, LC_MESSAGES.
Reference: GNU gettext API
1. Create source file “gettext_test.py”
The argument ‘myapplication’ is domain and ‘locale_dir’ is the directory where mo file is.
import gettext gettext.bindtextdomain('myapplication', 'locale_dir') gettext.textdomain('myapplication') _ = gettext.gettext print (_('Hello World!'))
2. Create <domain>.pot file with pygettext.py that is in Python<version>\Tools\i18n directory. The pot file myapplication.pot will be generated in the directory “locale_dir”.
python Python<version>\Tools\i18n\pygettext.py -d myapplication -p locale_dir gettext_test.py
The following is generated myapplication.pot.
# SOME DESCRIPTIVE TITLE. # Copyright (C) YEAR ORGANIZATION # FIRST AUTHOR <EMAIL@ADDRESS>, YEAR. msgid "" msgstr "" "Project-Id-Version: PACKAGE VERSION\n" "POT-Creation-Date: 2020-07-20 01:35+0900\n" "PO-Revision-Date: YEAR-MO-DA HO:MI+ZONE\n" "Last-Translator: FULL NAME <EMAIL@ADDRESS>\n" "Language-Team: LANGUAGE <LL@li.org>\n" "MIME-Version: 1.0\n" "Content-Type: text/plain; charset=cp932\n" "Content-Transfer-Encoding: 8bit\n" "Generated-By: pygettext.py 1.5\n" #: gettext_test.py:7 msgid "Hello World!" msgstr ""
3. Create po file and put it in the language directory.
Change the charset into utf-8, input the translation and save it chadnged myapplication.po. In my case, the Japanese “こんにちは世界!” that means “Hello World!” is put in msgstr.
# SOME DESCRIPTIVE TITLE. . . . "Content-Type: text/plain; charset=utf-8\n" . . . #: gettext_test.py:7 msgid "Hello World!" msgstr "こんにちは世界!"
Put the chadnged myapplication.po in the <directory_name>/language/LC_MESSAGES. The language is <language codes>_<country codes>. I put it in locale_dir/ja_JP/LC_MESSAGES.
The list of language code and country code: ISO-3166 Country Codes and ISO-639 Language Codes
4. Generate mo file from po file with Python<version>\Tools\i18n\msgfmt.py
python Python<version>\Tools\i18n\msgfmt.py myapplication.po
This image is the file construction.
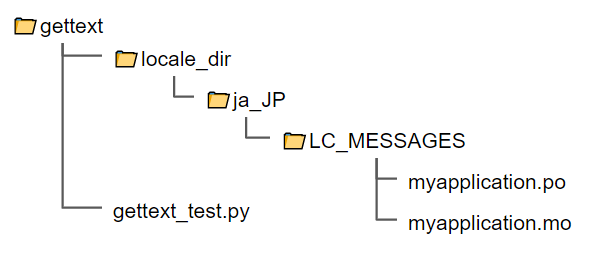
5. Execute gettext_test.py
python gettext_test.py こんにちは世界!
Method 2. Class-based API
This can be used in linux, unix and Windows.
Reference: Class-based API
1. Create source file “gettext_test.py”
Use translation(). The argument ‘myapplication’ is domain and ‘locale_dir’ is the directory where mo file is.
import os import gettext _ = gettext.translation( domain='myapplication', localedir = 'locale_dir', languages=['ja_JP'], fallback=True).gettext print (_('Hello World!'))
The rest processes are same as “Method 1. GNU gettext API” above.
2. Create <domain>.pot file with pygettext.py
3. Create po file and put it in the language directory <directory_name>/language/LC_MESSAGES.
4. Generate mo file from po file with Python<version>\Tools\i18n\msgfmt.py
5. Execute gettext_test.py
python gettext_test.py こんにちは世界!