First PySide Application
GOAL
To understand Qt and PySide(PySide2) and create PySide application that
Emvironment
WIndows10
Python2.7.8
*I use python2 because python3 can’t be used in Maya, but I think python3 is better for general application development. And PySide2 which is used in Maya2017~ is easy to use in python3.
What is Qt?
Qt is a cross-platform application framework with many modules providing services like network abstraction and XML handling, along with a very rich GUI package.
Though Qt is developed in C++, it can be used in Python, Java, Perl and so on with API. The application developed with Qt can be executed in any platform such as Windows, Linux, macOS, desktop and mobile.
Qt has been upgraded constantly, and the latest version in 2020 is Qt5.
What is PySide?
PySide is the python module as a Python binding of Qt. Functions, variables and modules of Qt can be used in Python via PySide. GUI application can be developed on multi platform, windows, Linux and macOS using Python with PySide.
PySide and Pyside2
PySide2 is upgraded PySide. PySide2 provides access to the Qt 5 framework while PySide provide access to Qt4 or below. Qt4 and PySide can be used in Maya2016 and before, Qt5 and PySide2 is used in Maya2017 and after.
If you are using python2, it’s easy to install PySide by using pip install, but you should build PySide2 by yourself. So If you want to use PySide2, please use python3 and its pip install.
The difference between PySide and PyQt
PyQt is also one of the python modules as a Python binding of Qt. The document “Differences Between PySide and PyQt” is clear and detailed. And “PyQt vs Pyside” is easy to understand their advantages and disadvantages.
Method
The following is the method to create your first PySide application. This is for PySide so please replace words for PySide2 if you need.
Install PySide
Open Command prompt and input pip command. In my case, ‘pip2’ is used because python3 is installed and the command just ‘pip’ is equal to pip3.
> pip2 install pyside
Check if PySide is successfully installed.
>py -2 Python 2.7.8.... >>> import PySide >>> PySide.__version__ '1.2.4' >>>
Hello World
This is an application program to display HelloWorld.
import sys from PySide.QtCore import * from PySide.QtGui import * #Create QApplication firstApp = QApplication(sys.argv) # Create a Label label = QLabel("Hello World") label.show() # Enter Qt application main loop firstApp.exec_() sys.exit()
Execute and small window will appear.
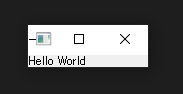
QtGui
QtGui module contains classes that control widgets of PySide. Widget is a UI component that is used in GUI application such as button, label, Matrix, Layout and so on. You can see all class in PySide.QtGui.
Create Widget
You can create original widget as a Class. Add single widgets into QVBoxLayout.
import sys from PySide.QtCore import * from PySide.QtGui import * class MyWidget(QWidget): def __init__(self): super(MyWidget, self).__init__() self.text = QLabel("Say Hello") self.text.setAlignment(Qt.AlignCenter) self.edit = QLineEdit("who?") self.button = QPushButton("Click here") self.output = QLabel("") self.layout = QVBoxLayout() self.layout.addWidget(self.text) self.layout.addWidget(self.edit) self.layout.addWidget(self.button) self.layout.addWidget(self.output) self.setLayout(self.layout) self.button.clicked.connect(self.sayHello) def sayHello(self): self.output.clear() self.output.setText("Hello " + self.edit.text()+"!") if __name__ == '__main__': app = QApplication(sys.argv) widget = MyWidget() widget.resize(300, 100) widget.show() widget.setWindowTitle("My Widget Test") sys.exit(app.exec_())
This is the window. Input name into line edit and click the button.
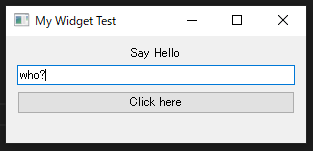
Then name you enter in the field is displayed.
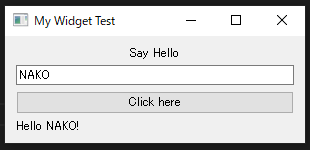