Why does category list return “Uncategorized”?
Problem
I’d like to display the list of all categories in my theme, but it return “Uncategorized”. The PHP code in index,php is as below.
<h1>Categories</h1> <?php $categories = get_the_category(); if ( $categories ) { foreach( $categories as $category ) { $output .= '<a href="' . get_category_link($category->term_id) . '">' . $category->cat_name . '</a>' . ' '; } echo $output; } ?>
The result is the following.
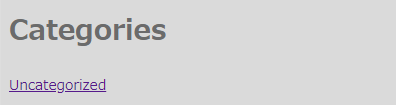
The cause of this problem
get_the_category(); is the function to get the categories of “current” post or page. This page is index.php with no category, so it return “Uncategorized”.
Solution
Use get_categories(); instead of get_the_category();.
<h1>Categories</h1> <?php $categories = get_categories(); if ( $categories ) { foreach( $categories as $category ) { $output .= '<a href="' . get_category_link($category->term_id) . '">' . $category->cat_name . '</a>' . ' '; } echo $output; } ?>
This is the result.
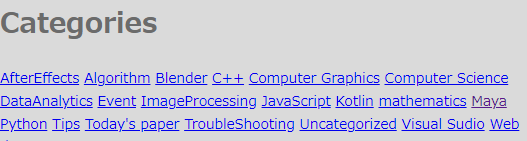