Create single.php for original theme
This is one of the articles about project “Create Original Theme in WordPress”.
GOAL
To create single.php
Environment
WordPress 5.5.1
XAMPP 7.4.10
single.php
<?php /** *this is the single post template *@package WordPress *@subpackage Techblog *@since Techblog 1.0 */ get_header(); ?> <div class="container"> <main id="main" class="site-main"> <?php while ( have_posts() ) : the_post(); get_template_part( 'content/content', 'single' ); if ( comments_open() || '0' != get_comments_number() ) : comments_template(); endif; endwhile; ?> </main> <?php get_sidebar();?> </div> <?php get_footer(); ?>
content/content-single.php
<?php /** * The template used for displaying single post content in single.php *@package WordPress *@subpackage Techblog *@since Techblog 1.0 */ ?> <article id="post-<?php the_ID(); ?>" <?php post_class(); ?>> <div class="main-content"> <div class="page-item"> <h1 class="entry-title"><?php the_title(); ?></h1> <div class="post-info"> <span class="sns"><a href="https://twitter.com/share" data-url="<?php the_permalink(); ?>" data-text="<?php echo get_the_title(); ?>" class="twitter-share-button" data-via="nako_new_high" data-show-count="false">Tweet</a><script async src="https://platform.twitter.com/widgets.js" charset="utf-8"></script></span><br/> <span class="post-date"><?php the_date();?></span> <?php $post_categories = get_the_category(); if ( $post_categories ) { foreach( $post_categories as $category ): ?> <span class= "post-categories"><a class="category" href= "<?php echo get_category_link($category->term_id) ?>" > <?php echo $category->cat_name ?> </a></span> <?php endforeach; ?> <?php }; ?> <br/> <?php if ( has_tag() ) : ?> <?php $tags = get_the_tags( get_the_ID() ); foreach ( $tags as $tag ) { echo '<span class= "post-tags"><a href="'. get_tag_link( $tag->term_id ) . '">#' . $tag->name . '</a></span>'; } ?> <?php endif; ?> </div> <?php the_content(); ?> </div> </div> </article>
Functions
has_tag()
This return true if the current post has tags.
get_the_tags( get_the_ID())
get_the_tags() retrieves the tags for the post that takes the id of the post as an argument. get_the_ID() retrieve the ID of the current item.
The tag objects can be retrieved by using for loop.
get_tag_link()
This function retrieves the link to the tag. It gets tag ID or object as an argument.
Result
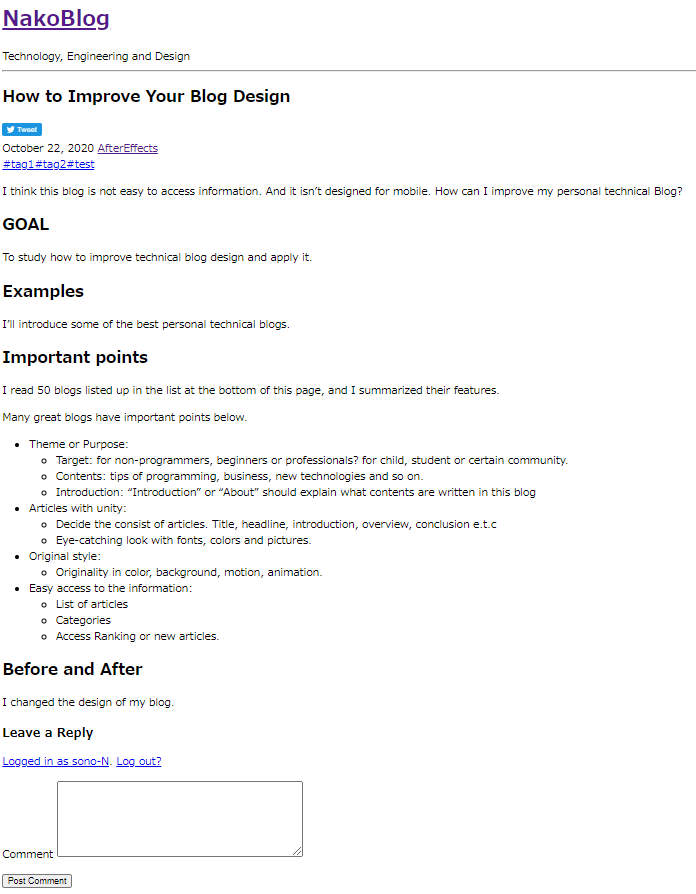
Change the style
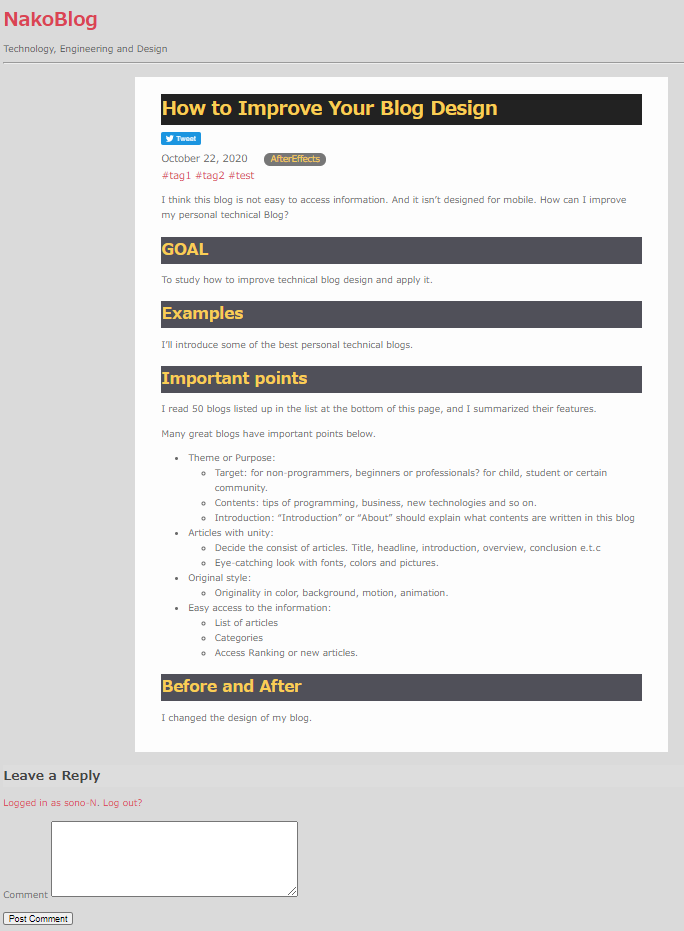