Create index.php for original theme
This is one of the articles about project “Create Original Theme in WordPress”.
GOAL
To create index.php
Environment
WordPress 5.5.1
XAMPP 7.4.10
index.php
<?php /** *This is main file for "techblog" theme *@package WordPress *@subpackage Techblog *@since Techblog 1.0 */ get_header(); ?> <div class="container"> <main id="main" class="site-main"> <div class= "main-content"> <h1>Recent Posts</h1> <?php $lastposts = get_posts( array( 'posts_per_page' => 3 ) ); if ( $lastposts ) { foreach ( $lastposts as $post ) : setup_postdata( $post ); ?> <div class="post-item recent-post"> <h2 class="entry-title"><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2> <div class="post-info"> <span class="post-date"><?php the_date();?></span> <?php $post_categories = get_the_category(); if ( $post_categories ) { foreach( $post_categories as $category ): ?> <span class= "post-categories"><a class="category" href= "<?php echo get_category_link($category->term_id) ?>" > <?php echo $category->cat_name ?> </a></span> <?php endforeach; ?> <?php }; ?> </div> <?php the_excerpt(); ?> </div> <?php endforeach; wp_reset_postdata(); }?> <h1>Categories</h1> <?php $categories = get_categories(); if ( $categories ) { foreach( $categories as $category ): ?> <a class="index category" href= "<?php echo get_category_link($category->term_id) ?>" > <?php echo $category->cat_name ?> </a> <?php endforeach; ?> <?php }; ?> </div> </main> <?php get_sidebar(); ?> </div> <?php get_footer(); ?>
Functions
get posts
$lastposts = get_posts( array('posts_per_page' => 3) );
loop the posts
if ( $lastposts ) { foreach ( $lastposts as $post ) : setup_postdata( $post ); ?> HTML is here <?php endforeach; ?>
This is equal to the following.
if ( $lastposts ) { foreach ( $lastposts as $post ) { setup_postdata( $post ); // Do something here } }
get the url of each post that is “root/<year>/<month>/<day>/<title>/” by default.
the_title(); // works in the loop
get the title of each post
the_date(); //works in the loop
get the date when the post is posted
the_date(); //works in the loop
get the categories of current post
$post_categories = get_the_category();
loop the categories
if ( $post_categories ) { foreach( $post_categories as $category ): ?> HTML is here <?php endforeach; ?> <?php }; ?>
This is equal to the following.
if ( $post_categories ) { foreach( $post_categories as $category ){ HTML is here } }
get the url of the category that is “root/category/<category name>/” by default
get_category_link($category->term_id)
get the category name from $category
$category->cat_name
get the excerpt of the post
the_excerpt();
How to change the length of the excerpt
Add filter in the functions.php. If you’d like to know about add_filter(), please check the article “What is Hook in WordPress?“.
function twpp_change_excerpt_length( $length ) { return 20; } add_filter( 'excerpt_length', 'twpp_change_excerpt_length', 999 );
get all categories of the site
$categories = get_categories();
Result
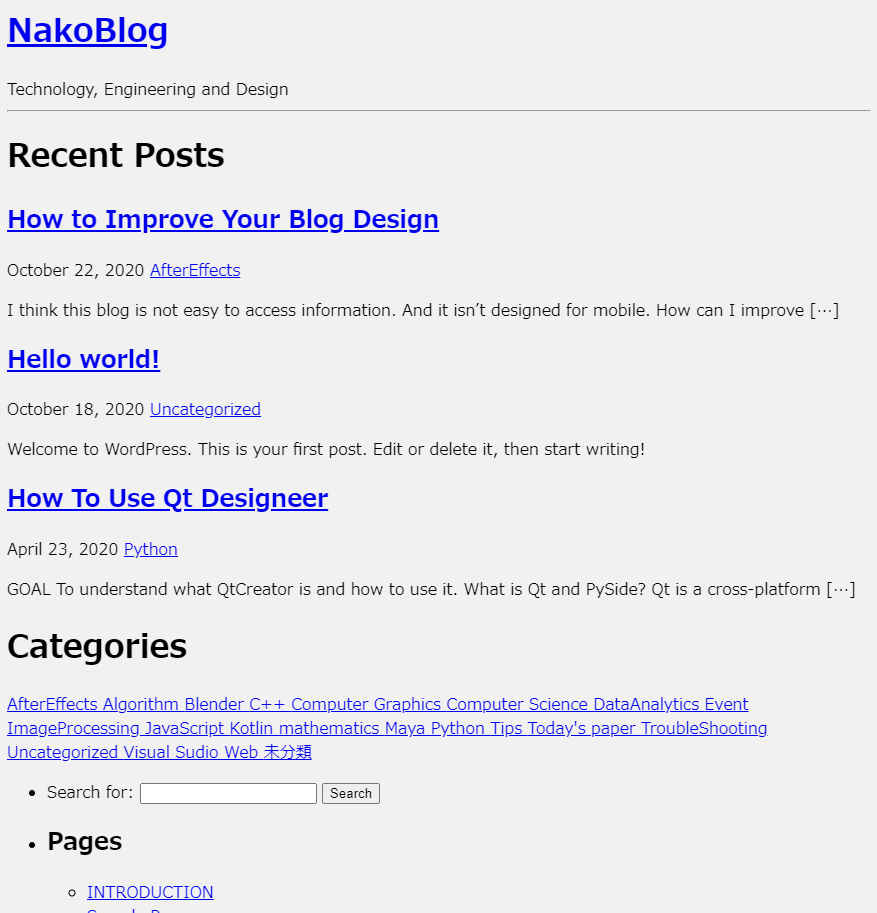
Change style
I customized the design of the page by editing style.css.
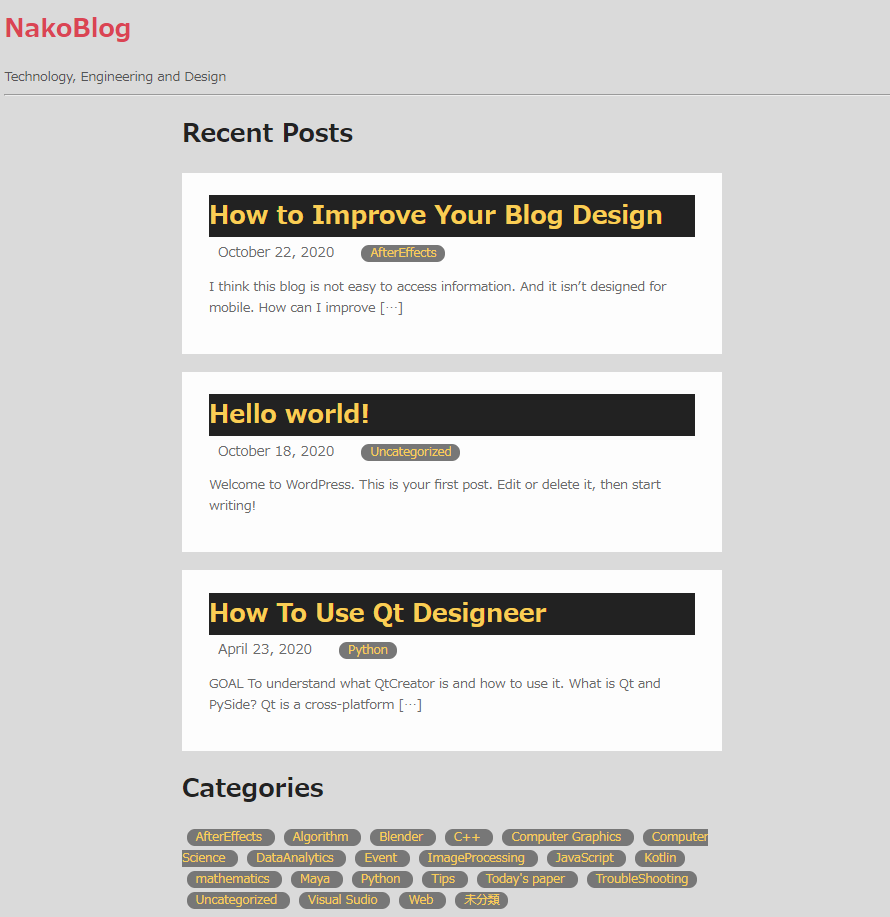