What is Hook in WordPress?
GOAL
To understand what is the hook in WordPress and how to use it.
Environment
WordPress 5.5.1
What the hook is in WordPress
Hooks are a way for one piece of code to interact/modify another piece of code at specific, pre-defined spots.
from Plugin Handbook in wordpress.org
When the specific event occurs, the registered function is hooked.
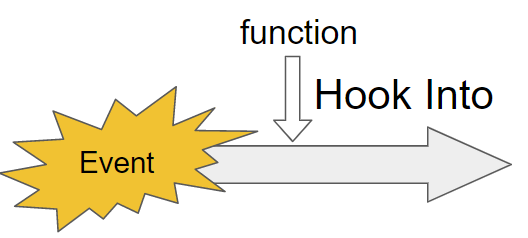
For example, if you define the function addName() and add it to the hook ‘the_content’, you can add name to the content of the article.
$name = 'Nako'; function addName($content) { return $content .'('.$name.')'; } add_filter('the_content', 'addName');
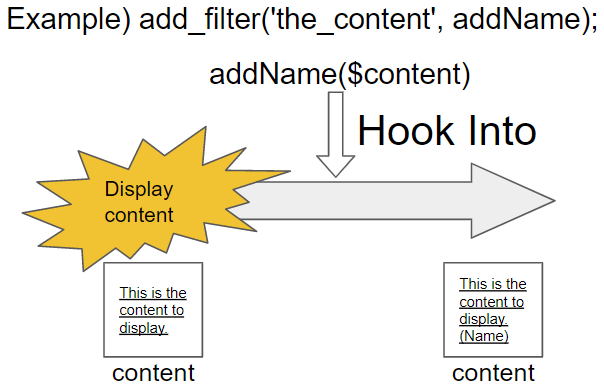
Add filter and action
There are 2 types of hook, filter and action. You can see the list of filters in Plugin API/Filter Reference and the list of actions in Plugin API/Action Reference.
The filter hooks are defined by apply_filters() and add function to the filter hook by add_filter(). And the action hools are defined by do_action() and add action to the action hook by add_action().
The following is the outline diagram of apply_filters, do_action, add_action and add_filter.
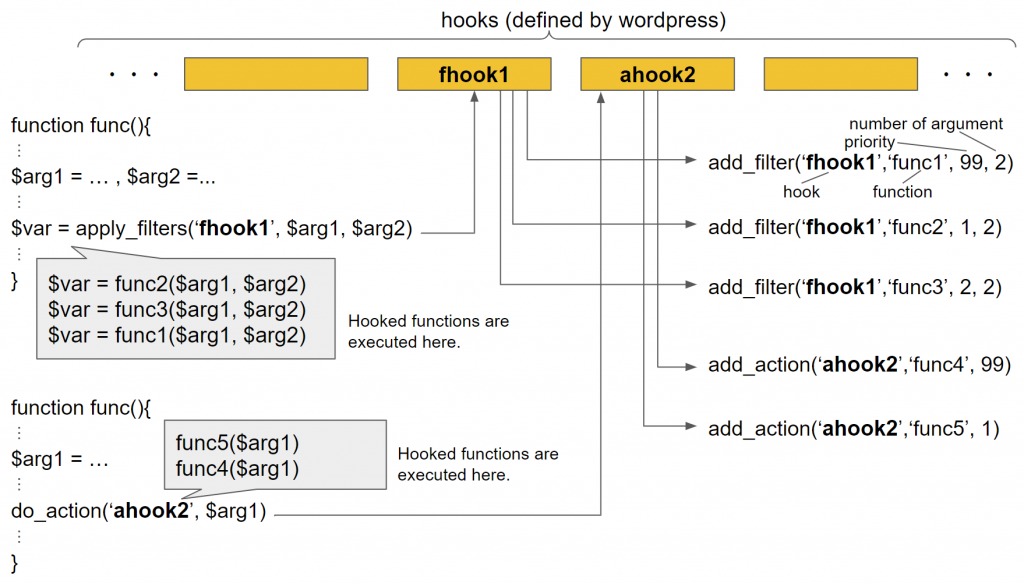
Example
For example, this is one of the apply_filters functions in post-template.php.
function the_content( $more_link_text = null, $strip_teaser = false ) { $content = get_the_content( $more_link_text, $strip_teaser ); $content = apply_filters( 'the_content', $content ); $content = str_replace( ']]>', ']]>', $content ); echo $content; }
The difference between filters and actions
Action is used to do some user-defined action, but on the other hands filter is used to rewrite or return the variable. So the function added by add_filter() has always return value.