How to Display Custom Property on Properties Editor in Blender Addon
GOAL
Today’s goal is to develop an addon and display custom property on Properties editor. For example, add “target_obj” property to bpy.types.Object for management and edit the value of “target_obj” with Properties editor.
Environment
Blender2.83(LTS)
Windows10
Method
1. Register property
The following is the code of addon just add the property “target_obj” to bpy.types.Object.
bl_info = { "name": "Add Target Property", "description": "test addon to add target_obj properties to Object", "version": (1, 0), "blender": (2, 80, 0), "category": "3D View", } import bpy def register(): bpy.types.Object.target_obj = bpy.props.PointerProperty(name="TargetObj", type=bpy.types.Object) def unregister(): del bpy.types.Object.target_obj if __name__ == '__main__': register()
At this time, the property “target_obj” is not displayed in Properties Editor.
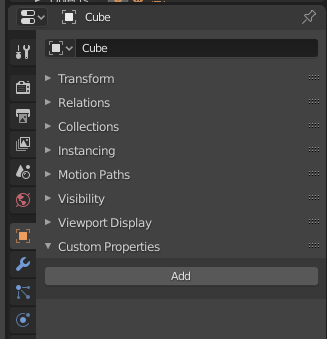
However, users can access the property by python script.
>>> print(bpy.context.object.target_obj) None >>> bpy.context.object.target_obj = bpy.data.objects["Cone"] >>> print(bpy.context.object.target_obj) <bpy_struct, Object("Cone")>
And it is necessary to access the property once in order to display it in properties editor.
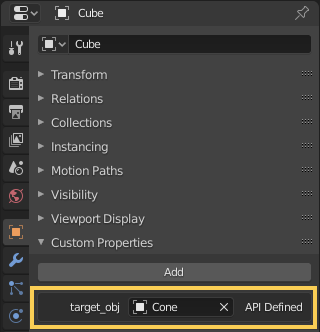
2. Access the property
To display the custom property in properties editor, access the property in addon.
Use handler to call the function to set value. The following is complete code.
bl_info = { "name": "Add Target Property", "description": "test addon to add target_obj properties to Object", "version": (1, 0), "blender": (2, 80, 0), "category": "3D View", } import bpy from bpy.app.handlers import persistent @persistent def obj_init(scene): if hasattr(bpy.context, "object") and not bpy.context.object.target_obj: bpy.context.object.target_obj = None bpy.app.handlers.depsgraph_update_post.append(obj_init) def register(): bpy.types.Object.target_obj = bpy.props.PointerProperty(name="TargetObj", type=bpy.types.Object) def unregister(): del bpy.types.Object.target_obj if __name__ == '__main__': register()