How To Add Customize Menu for Original WordPress Theme
This is one of the articles about project “Create Original Theme in WordPress”.
GOAL
Today’s goal is to add original menu to the “Theme Customizer” as below.
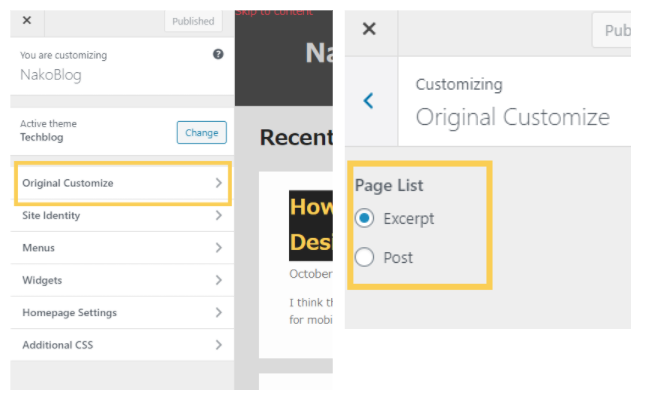
The Theme Customizer can be opened from “Appearance” > “Customize” in WordPress admin page.
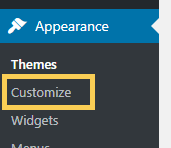
If the “Excerpt” is selected the excerpts of the post are displayed as page list and if the “Post” is selected the posts themselves are displayed as page list.
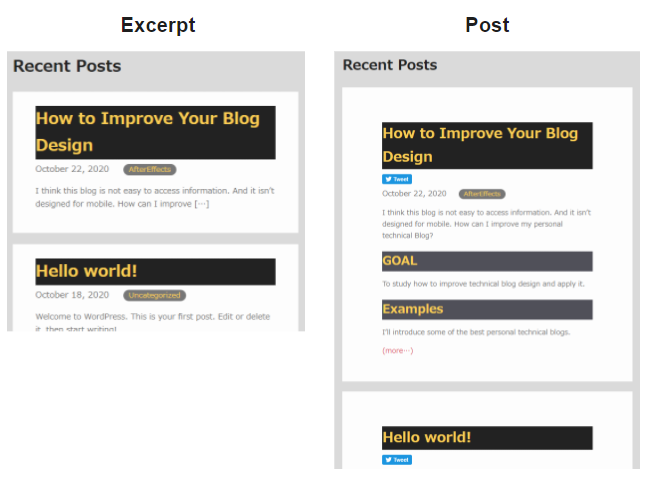
Environment
WordPress 5.5.1
XAMPP 7.4.10
Method
Reference: Theme Customization API in wordpress.org
1. Define customizer-register function
Add function to register new customizer to the hook “customize_register” in functions.php of your theme.
fucntion.php
function techblog_customize_register( $wp_customize ) { // add functions here } add_action( 'customize_register', 'techblog_customize_register' );
If you’d like to know about Hook in WordPress development, check “Hooks (wordpress.org)” or an article “What is Hook in WordPress?“.
2. Add section
Section is the group of customizer.
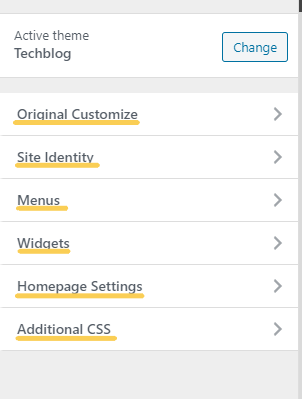
Use WP_Customize_Manager::add_section() function to add a new section.
wp_customize->add_section( 'original_customize', // id for customizer section array( 'title' => 'Original Customize', // the title of the section 'priority' => 10, ) );
We can also few built-in sections. In this case, we don’t need to declare them with add_section().The followings are built-in sections. (Refer to wordpress.org)
- title_tagline – Site Title & Tagline (and Site Icon in WP 4.3+)
- colors – Colors
- header_image – Header Image
- background_image – Background Image
- nav – Navigation
- static_front_page – Static Front Page
3. Add setting
Setting handles saving and sanitizing of settings of the theme. For example, setting has the color of the element.
Settings automatically use WordPress’s theme_mod features to get/set settings for your theme.
(from Adding a New Setting in wordpress.org)
WP_Customize_Setting determines settings of input control such as its type, default value or sanitize function. See the parameters of WP_Customize_Setting::__construct for details.
Use WP_Customize_Manager::add_setting function to add setting.
$wp_customize->add_setting( 'list_excerpt_setting', array( 'default' => 'excerpt', ) );
4. Add control
Control is used to render an input control on the Theme Customizer. There are some types as below. The default is text input.
Control type. Core controls include ‘text’, ‘checkbox’, ‘textarea’, ‘radio’, ‘select’, and ‘dropdown-pages’. Additional input types such as ’email’, ‘url’, ‘number’, ‘hidden’, and ‘date’ are supported implicitly.
(from WP_Customize_Control::__construct in wordpress.org)
Use WP_Customize_Manager::add_control with argument array to add control. In my case, I added radio button input control with 2 choices “Excerpt” and “Post”.
$wp_customize->add_control( 'list_excerpt_selection', array( 'settings' => 'list_excerpt_setting', 'label' => 'Page List', 'section' => 'original_customize', 'type' => 'radio', 'default' => 'excerpt', 'choices' => array( 'excerpt' => __( 'Excerpt' ), 'post' => __( 'Post' ), ), ) );
Complete code
function.php
/*------------------- Theme Customizer -------------------*/ function techblog_customize_register( $wp_customize ) { $wp_customize->add_section( 'original_customize', array( 'title' => 'Original Customize', 'priority' => 10, ) ); $wp_customize->add_setting( 'list_excerpt_setting', array( 'default' => 'excerpt', ) ); $wp_customize->add_control( 'list_excerpt_selection', array( 'settings' => 'list_excerpt_setting', 'label' => 'Page List', 'section' => 'original_customize', 'type' => 'radio', 'choices' => array( 'excerpt' => __( 'Excerpt' ), 'post' => __( 'Post' ), ), ) ); } add_action( 'customize_register', 'techblog_customize_register' );
How to get the value of customizer.
We can get the value of customizer with get_theme_mod() function. In this case the argument $name is the name of added setting.
index.php
$use_excerpt = get_theme_mod('list_excerpt_setting') == 'excerpt'; if ( $lastposts ) { foreach ( $lastposts as $post ) : setup_postdata( $post ); if ($use_excerpt){ ?> <?php the_excerpt(); ?> . . . <?php } else{ ?> <div class="post-item recent-post"> <?php the_post(); ?>
Result
The new section and control are displayed in Theme Customizer.
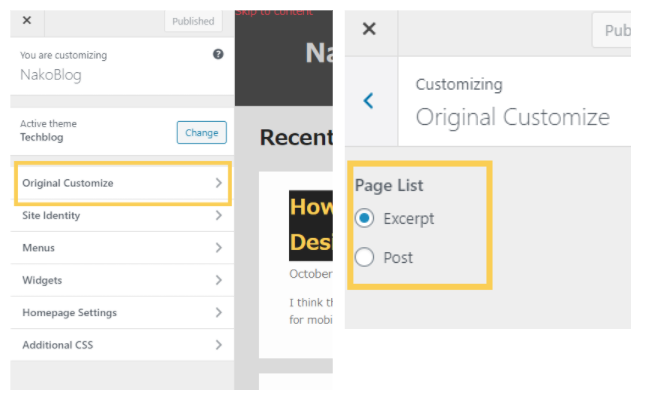