How to detect the child widget destroy?
GOAL
To do some functions when the child widget is deleted.
Environment
Windows 10
Python 3.8.7
PySide2 5.15.2
Method
Connect the function to child’s signal “destroyed” as below.
import sys from PySide2.QtWidgets import * class ItemWidget(QWidget): def __init__(self, id_str="", parent=None): super(ItemWidget, self).__init__(parent) self._generateUI() def _generateUI(self): main_layout = QGridLayout() self.setLayout(main_layout) title = QLabel("title") main_layout.addWidget(title, 0, 0, 1, 3) close_button = QPushButton("×") close_button.setFixedWidth(30) close_button.clicked.connect(self._close_widget) main_layout.addWidget(close_button, 0, 3, 1, 1) def _close_widget(self): self.deleteLater() class MyMainWindow(QMainWindow): def __init__(self, parent=None): super(MyMainWindow, self).__init__(parent) self._generateUI() def _generateUI(self): main_widget = QWidget() self.main_layout = QVBoxLayout() main_widget.setLayout(self.main_layout) self.setCentralWidget(main_widget) item = ItemWidget(str(self.id_num)) item.destroyed.connect(self._deletedItemFunc) # connect the function to execute self.main_layout.addWidget(item) def _deletedItemFunc(self): print("deleted") # when the item deleted, the message "deleted" is displayed def launch(): app = QApplication.instance() if not app: app = QApplication(sys.argv) widget = MyMainWindow() widget.show() app.exec_() launch()
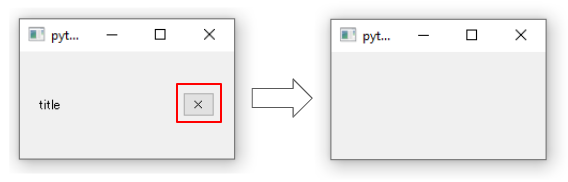
When click × button, the child widget deleted and the message “deleted” is displayed.
Appendix
If the child is removed from the parent layout. You can add function at the same time.
self.main_layout.removeWIdget(item) self._deletedItemFunc()