How to use posted data in the same page
GOAL
To post the input data and display it on the same page with PHP.
Environment
Windows10
XAMPP 7.4.10
Preparation
1. Change the PHP program
Create a new PHP file “index.php” to submit data. You can see the details of this program in “How To Run PHP in XAMPP“.
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <title>test</title> </head> <body> <form action="posted.php" method="POST"> <label>Input message:</label> <input type="text" name="message" /> <input type="submit" value="Submit!" /> </form> </body> </html>
2. Set action = “”
The action specifies the file to call. If you won’t move the page, action should be “”.
Method
Method 1. Use $_SERVER[‘REQUEST_METHOD’]
$_SERVER[‘REQUEST_METHOD’] is the variable to store the requested method. It returns the called requested method when the page is requested.
<body> <?php if ($_SERVER['REQUEST_METHOD'] === 'POST') { echo "The input data is ".$message; } ?> <form action="" method="POST"> <label>Input message:</label> <input type="text" name="message" /> <input type="submit" value="Submit!" /> </form> </body>
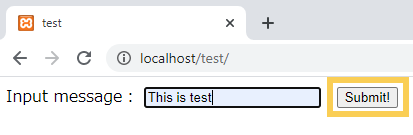
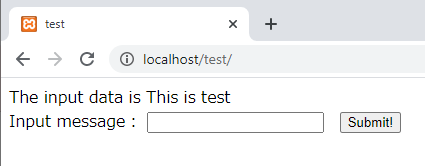
Method 2. Use $_POST directly
<body> <?php if ($_POST) { echo "The input data is ".$_POST['message']; } ?> <form action="" method="POST"> <label>Input message:</label> <input type="text" name="message" /> <input type="submit" value="Submit!" /> </form> </body>
The result is the same as above.
Method 3. Use isset($_POST[‘message’])
<body> <?php if ($_POST) { echo "The input data is ".$_POST['message']; } ?> <form action="" method="POST"> <label>Input message:</label> <input type="text" name="message" /> <input type="submit" value="Submit!" /> </form> </body>
The result is the same as above. The strong point of this method is that the existence of each keys of $_POST can be determined.