How To Use Qt Designeer
GOAL
To understand what QtCreator is and how to use it.
What is Qt and PySide?
Qt is a cross-platform application framework with many modules providing services like network abstraction and XML handling, along with a very rich GUI package. Check the article “First PySide Application” for details of Qt and PySide.
What is Qt Designer?
Qt Designer is the Qt tool for designing and building GUIs with Qt Widgets. Qt Designer makes it easy to layout UI widgets with PySide.
Reference: Qt Designer Manual
How to use
Environment
Windows10
Python3.8.5
PySide2-5.15.1
Install and Start Qt Designer
1. Install PySide or PySide2
pip install PySide2 Collecting PySide2 Downloading PySide2-5.15.1-5.15.1-cp35.cp36.cp37.cp38.cp39-none-win_amd64.whl (136.9 MB) |████████████████████████ | 101.6 MB 1.7 MB/s eta 0:00:22 ... omitted ... Successfully installed PySide2-5.15.1 shiboken2-5.15.1
Use pip3 if you need. When you use Python2, PySide2 can’t be installed by pip command, so please install python3 or use PySide.
2. Start designer.exe
Start PythonDir\site-packages\PySide2\designer.exe
You can find the directory “PySide2” by PySide2.__file__
import PySide2 print(PySide2.__file__)
Or you can find the python directory with the command below.
C: >where python
If you are using Python2, designer.exe exists in Python2\Lib\site-packages\PySide directory.
Create Dialog
1. Create a new form
When you start the designer.exe, the “New Form” window will open automatically. If not, click File > New
Select any template you like and click the button “Create”.
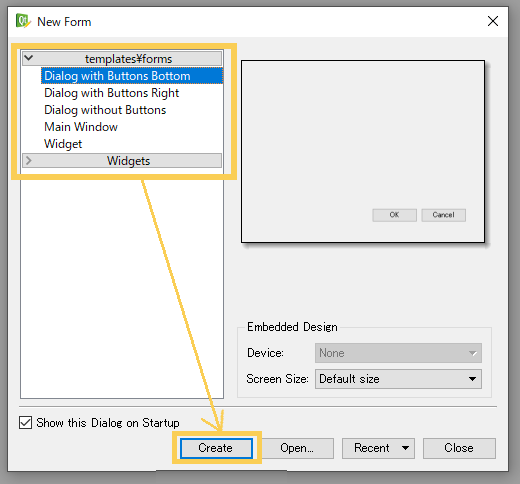
An empty untitled widget with “OK” and “Cancel” buttons will appear.
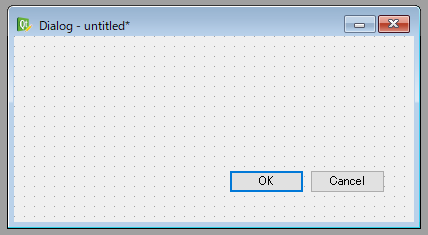
2 Put the widget on the empty Dialog
Drag & Drop any widget you like from “Widget Box” and put it into the empty Dialog. I created a dialog to export reduced object in DCC tool.
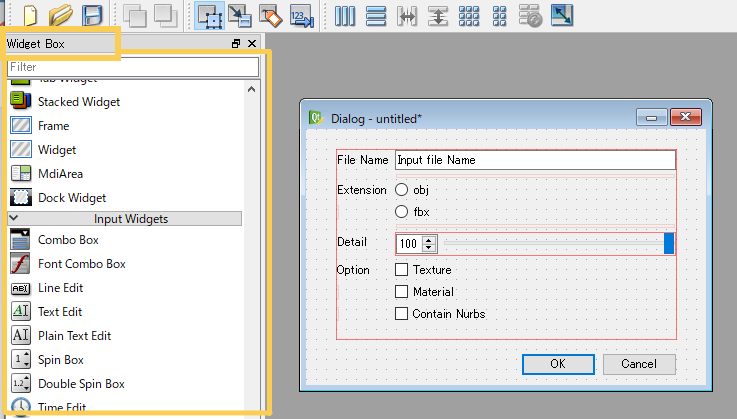
The construction of this form is the following.
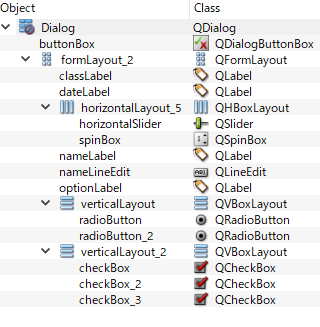
And reform the widgets in main layout. Right-click on the form outside of the form Layout and click layout>Lay Out Vertically.
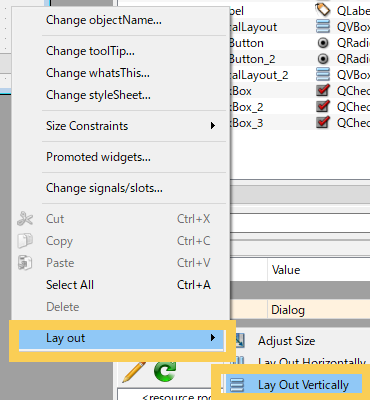
The widgets are arranged at even intervals in the main layout.
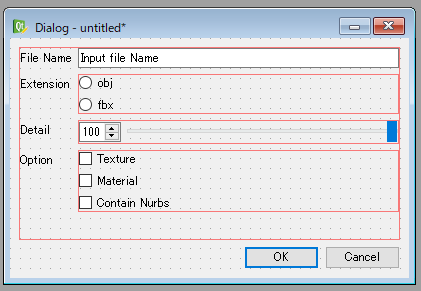
Set the default values and change settings of each property with “Property Editor.”
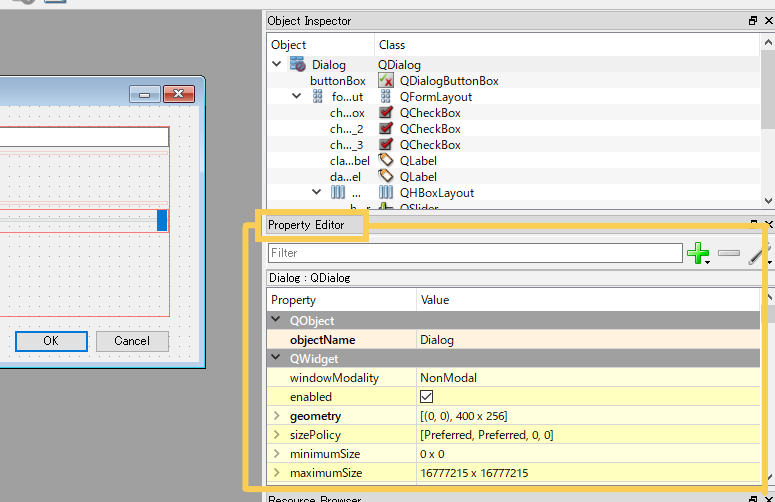
3. Connect the signals to the slots
In Qt Designer, you can connect the signal of the widget to the slot of another widget, in other words connect some widgets as input and output. In this case, Spin Box and Horizontal Slider should move in conjunction with each other.
Change the mode to edit signals/slots by clicking Form>Edit Signals/Slots.
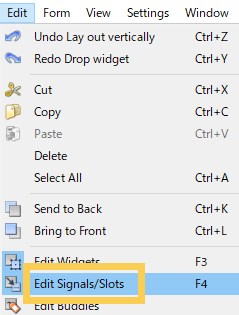
Drag & drop from Spin Box to Horizontal Slider.
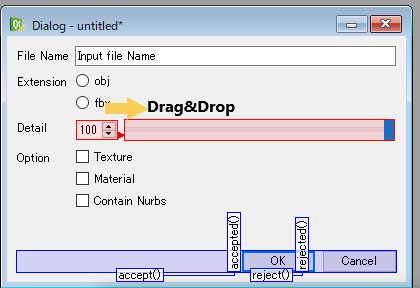
The signal is valueChanged and the connected slot is setValue.
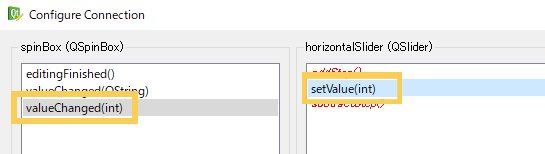
In contrast, drag & drop from Horizontal Slider to Spin Box and connect them in the same way.
4. Preview
Preview the form you created by Form>Preview… and check if it works well.
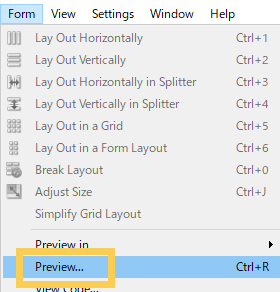
5. Save the UI as Designer UI files (.ui)
Save the dialog you created as “reduced_export.ui”.
Load .ui from Python file
You can load .ui file you created from python file.
Run the program below and the dialog you created will be displayed.
# -*- coding: utf-8 -* import sys from PySide2 import QtWidgets from PySide2.QtUiTools import QUiLoader if __name__ == '__main__': app = QtWidgets.QApplication(sys.argv) loader = QUiLoader() widget = loader.load('reduced_export.ui', None) if not widget: print(loader.errorString()) sys.exit(-1) widget.show() sys.exit(app.exec_())
Or define ExportUI class that inherits QMainWindow.
class ExportUI(QtWidgets.QMainWindow): def __init__(self, parent=None): super(ExportUI, self).__init__(parent) loader = QUiLoader() self.ui = loader.load('reduced_export.ui') if not self.ui: print(loader.errorString()) sys.exit(-1) print("OPEN") def getUi(self): return self.ui if __name__ == '__main__': app = QtWidgets.QApplication(sys.argv) exportUi = ExportUI() widget = exportUi.getUi() widget.show() sys.exit(app.exec_())
Connect functions you defined to the widgets
When the “OK” button is clicked, or the buttonBox widget is accepted, call the function export() that is the function to export the selected object in DCC tools. You can connect them by using “self.ui.buttonBox.accepted.connect(self.export)”.
In this example the function export() is omitted.
# -*- coding: utf-8 -* import sys from PySide2 import QtWidgets from PySide2.QtUiTools import QUiLoader def exportObj(name, extension, detail, options): # omitted the function to export object ext = 'fbx' if extension else 'obj' print(f"exported {name}.{ext} with {detail}% detail and option {options}.") class ExportUI(QtWidgets.QMainWindow): def __init__(self, parent=None): super(ExportUI, self).__init__(parent) loader = QUiLoader() self.ui = loader.load('reduced_export.ui') if not self.ui: print(loader.errorString()) sys.exit(-1) print("OPEN") self.ui.buttonBox.accepted.connect(self.export) def getUi(self): return self.ui def export(self): name = self.ui.nameLineEdit.text() extension = 0 if self.ui.radioButton.toggle() else 1 detail = self.ui.horizontalSlider.value() options = [self.ui.checkBox.isChecked(), self.ui.checkBox_2.isChecked() ,self.ui.checkBox_3.isChecked()] exportObj(name, extension, detail, options) if __name__ == '__main__': app = QtWidgets.QApplication(sys.argv) exportUi = ExportUI() widget = exportUi.getUi() widget.show() sys.exit(app.exec_())
You should know the classes and functions to get values such as QnameLineEdit.text() or QRadioButton.isChecked(). And you can see the class and the name of each widget in Property Editor of Qt Designer.
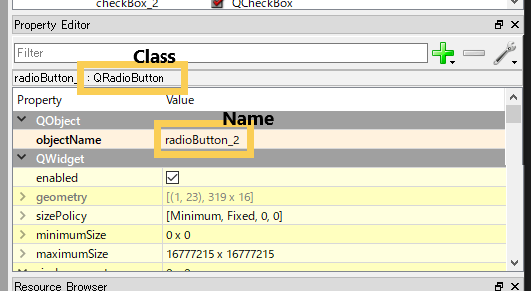
Run
Run the python code above and set the values on the displayed dialog.
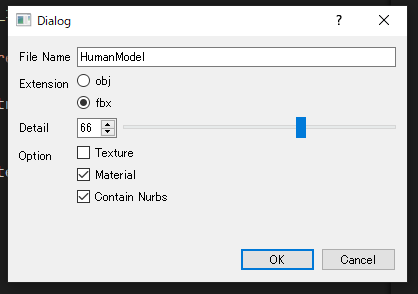
Click the “OK” button. Then the message below will be displayed on the console.
exported HumanModel.fbx with 66% detail and option [False, True, True].
Other tools for Qt programming
QtCreator
QtCreator is C++ IDE for development with Qt. It has an editor with useful functions, navigation tools, GUI tools for UI design and so on.
Reference: Qt Creator Manual
Qt Linguist
Qt Linguist is a tool for translating Qt C++ and Qt Quick applications into local languages.
Reference: Qt Linguist Manual
Qt Assistant
Qt Assistant is a tool for viewing on-line documentation for Qt programming.
Reference: Qt Assistant Manual