How To Get Mapping of UV and Vertex
GOAL
To get correspondence of UV coordinates and vertex id in the object in Blender scene by Blender Python. The following is output of the script.
uv: 0.625 0.5 vertex id: 0 uv: 0.875 0.5 vertex id: 4 uv: 0.875 0.75 vertex id: 6 uv: 0.625 0.75 vertex id: 2 uv: 0.375 0.75 vertex id: 3 . . .
Environment
Blender 2.83 (Python 3.7.4)
Windows 10
Method
Mesh Loop
Use Mesh Loop to get UV and vertex data.
In unfolded UV, the correspondence between the point of UV map and the vertex of 3D object is not one-to-one. Thus we use ‘Mesh Loop’ instead of vertex or edge to distinct point of UV map.
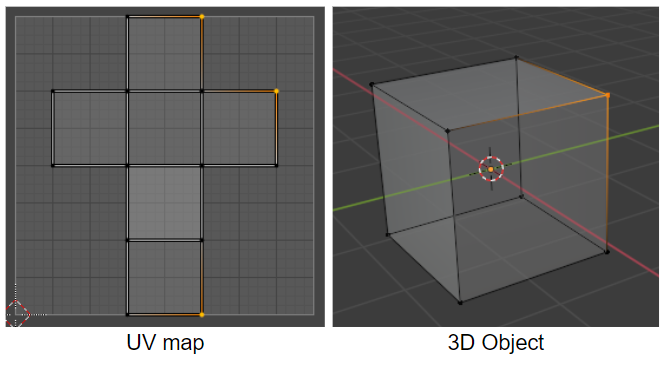
Mesh Loop is an element surrounding a face, which consists of edges and vertices. Each quad face consists of 4 Mesh Loops as below. For example, cube objects have 6face, 24 mesh loops.
import bpy obj = bpy.data.objects[obj_name] mesh_loops = obj.data.loops for mesh_loop in mesh_loops: print(mesh_loop)
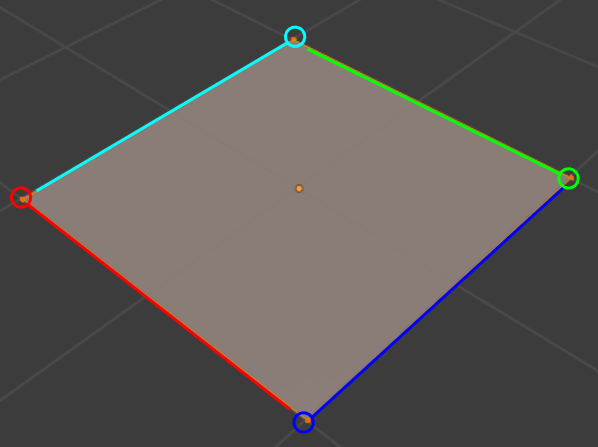
Mesh UV Loop
UVs have Mesh UV Loops as a counterpart to the Mesh Loops. And the correspondence between Mesh Loop and MeshUVLoop is one-to-one.
import bpy obj = bpy.data.objects[obj_name] mesh_uv_loop = obj.data.uv_layers[0].data[loop_index]
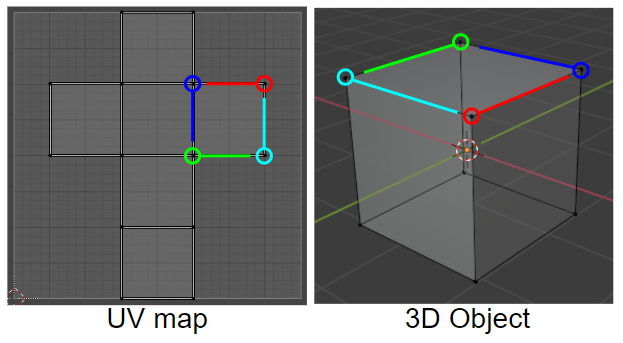
Source Code
import bpy def get_uvs(obj_name): obj = bpy.data.objects[obj_name] mesh_loops = obj.data.loops for i, mesh_loop in enumerate(mesh_loops): mesh_uv_loop = obj.data.uv_layers[0].data[i] print("uv:", mesh_uv_loop.uv[0], mesh_uv_loop.uv[1], "vertex id:", mesh_loop.vertex_index) get_uvs("Cube")