How To Use QInputDialog in PySide
GOAL
Today’s goal is to create a simple dialog by using QInputDialog in PySide.
Environment
Windows 10
Python 3.8.7
PySide2 (5.15.2)
Method
Reference: QInputDialog
Create the simplest dialog
There are 4 types of dialogs, getText, getInt, getDouble and getItem. Here is an example of QInputDialog() in https://gist.github.com/s-nako/1821d3c25997ec4a868f1e5f0bd2f687.
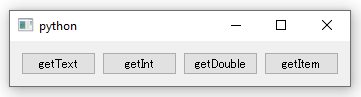
getText Dialog
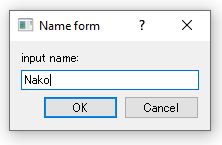
def show_get_text_dialog(self): name, ok = QInputDialog.getText(self, 'Name form', 'input name:') if ok: print("input is ", name)
getInt Dialog
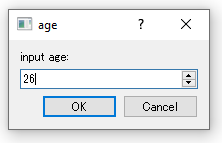
def show_get_int_dialog(self): age, ok = QInputDialog.getInt(self, "age", "input age:", minValue=0) if ok: print("input is ", age)
getDouble Dialog
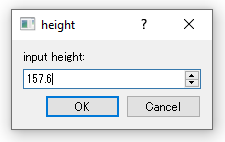
def show_get_double_dialog(self): height, ok = QInputDialog.getDouble(self, "height", "input height:", minValue=0) if ok: print("input is ", height)
getItem Dialog
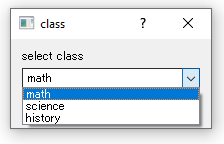
def show_get_item_dialog(self): selected_class, ok = QInputDialog.getItem(self, "class", "select class", ["math", "science", "history"]) if ok: print("input is ", selected_class)
Customize dialog
You can customize dialog with set functions as below.
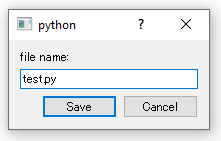
def show_save_dialog(self): dialog = QInputDialog() dialog.setOkButtonText("Save") dialog.setLabelText("file name:") dialog.setInputMode(QInputDialog.TextInput) ok = dialog.exec_() file_name = dialog.textValue() if ok: print(file_name + " was saved") else: print(file_name + " was not saved")