How To Create On/Off Button In PySide
GOAL
Today’s goal is to create On/Off button in my PySide UI as below.
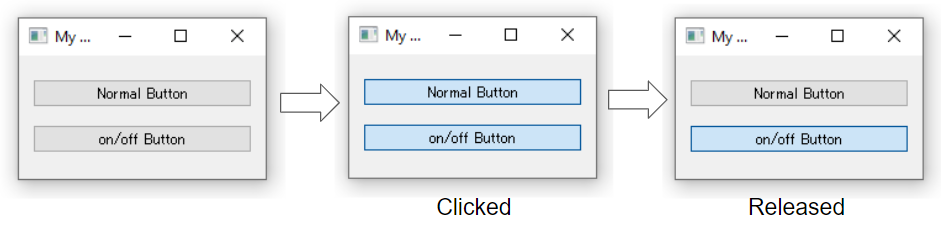
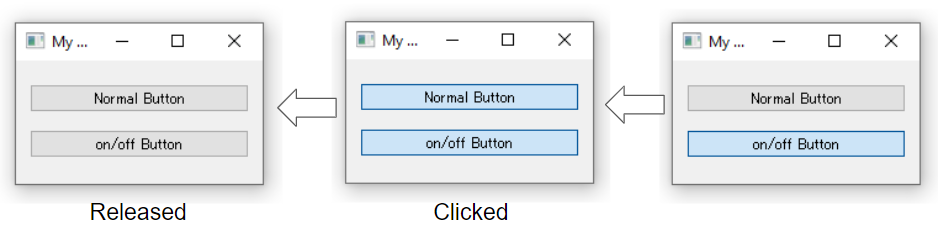
Environment
Windows 10
Python 3.8.7
PySide2 (5.15.2)
Method
1. Create a normal QPushButton
import sys from PySide2.QtWidgets import * class MyWidget(QWidget): def __init__(self): super(MyWidget, self).__init__() self.layout = QVBoxLayout() self.on_off_button = QPushButton("on/off Button") self.on_off_button.setCheckable(True) #self.layout.addWidget(self.on_off_button) self.setLayout(self.layout) if __name__ == '__main__': app = QApplication(sys.argv) widget = MyWidget() widget.show() sys.exit(app.exec_())
2. Use setCheckable() method
QAbstractButton has a method setCheckable().
class MyWidget(QWidget): def __init__(self): super(MyWidget, self).__init__() self.layout = QVBoxLayout() self.on_off_button = QPushButton("on/off Button") self.on_off_button.setCheckable(True) # set Checkable True self.layout.addWidget(self.on_off_button) self.setLayout(self.layout)
How to change the status, checked or unchecked
To change the status manually, use the setChecked() method of QAbstractButton.
For example, reset button can be implemented by using the function to set the button unchecked.
class MyWidget(QWidget): def __init__(self): super(MyWidget, self).__init__() self.layout = QGridLayout() self.reset_button = QPushButton("Reset") self.reset_button.clicked.connect(self._reset) self.layout.addWidget(self.reset_button,0,0,1,3) self.on_off_button1 = QPushButton("on/off 1") self.on_off_button1.setCheckable(True) self.layout.addWidget(self.on_off_button1,1,0,1,1) self.on_off_button2 = QPushButton("on/off 2") self.on_off_button2.setCheckable(True) self.layout.addWidget(self.on_off_button2,1,1,1,1) self.on_off_button3 = QPushButton("on/off 3") self.on_off_button3.setCheckable(True) self.layout.addWidget(self.on_off_button3,1,2,1,1) self.setLayout(self.layout) def _reset(self): self.on_off_button1.setChecked(False) self.on_off_button2.setChecked(False) self.on_off_button3.setChecked(False)
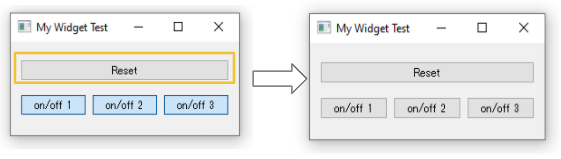