How To Run Python Script When Blender Starts
GOAL
To run Python script when Blender starts only once or every time.
Environment
Blender 2.83
Windows 10
Method
I created a simple Python script “Documents\blenderPython\test\spheres.py” to add spheres as below
import bpy for i in range(3): bpy.ops.mesh.primitive_uv_sphere_add(radius=1, location=((i-1)*3, 0, 3))
Only once
Start Blender with command
Start blender in command prompt with an argument -P or –python.
Reference: Python Options in Blender 2.91 Manual
blender -P Documents\blenderPython\test\spheres.py
The following is the result of startup scene.
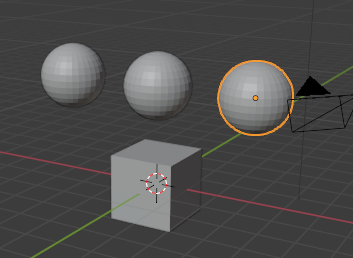
Every time
Method 1. Create bat file.
Create batch file and start Blender with it. Save the command above as “blender_start.bat” and execute it.
Method 2. Hook the function to handler
To run script every time, use handler to execute functions when blender starts. This method can be used in your Addon.
Reference: Application Handlers (bpy.app.handlers) in Blender2.91 Manual
startup_sphere.py
import bpy from bpy.app.handlers import persistent @persistent def add_sphere(dummy): for i in range(3): bpy.ops.mesh.primitive_uv_sphere_add(radius=1, location=((i - 1) * 3, 0, 3)) def register(): bpy.app.handlers.load_post.append(add_sphere) def unregister(): pass if __name__ == "__main__": register()
Put the file startup_sphere.py in the user startup directory such as C:\Users\<USER_NAME>\AppData\Roaming\Blender Foundation\Blender\2.83\scripts\startup. The files in the start up directory are executed when blender starts.
Reference: Script Loading in Blender Python API document
The following is the result of startup scene.
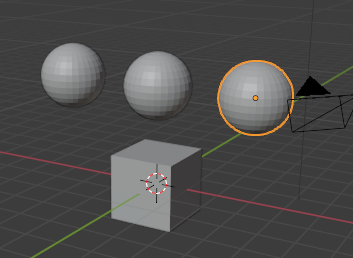
Wrong Example
You can’t put the python file that has operations of bpy.ops as below in user startup directory.
import bpy for i in range(3): bpy.ops.mesh.primitive_uv_sphere_add(radius=1, location=((i - 1) * 3, 0, 3))
import bpy from bpy.app.handlers import persistent def add_sphere(): for i in range(3): bpy.ops.mesh.primitive_uv_sphere_add(radius=1, location=((i - 1) * 3, 0, 3)) def register(): add_sphere() def unregister(): pass if __name__ == "__main__": register()
The error “AttributeError: ‘_RestrictContext’ object has no attribute ‘view_layer'” will occurs when the addon is activated. Check “[Blender Error] AttributeError: ‘_RestrictContext’ object has no attribute ‘view_layer’” for details.
You have a period on your import bpy
Thank you. I’ve corrected.