How To Insert Widget To The Layout Counting From The End
GOAL
To insert widget to the layout counting from the end. For example, I’d like to create the add button to add widget at the second position from the end as below.
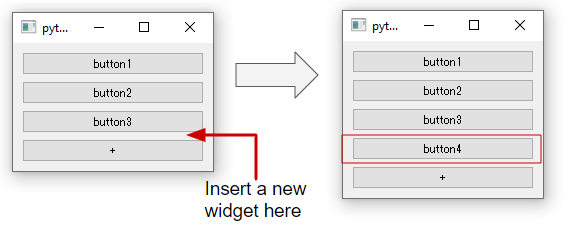
Environment
Windows 10
Python 3.8.7
PySide2 5.15.2
Method
At first, generate widget and set layout. I created a simple window as below.
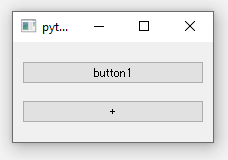
import sys from PySide2.QtWidgets import * class MyMainWindow(QMainWindow): def __init__(self, parent=None): super(MyMainWindow, self).__init__(parent) self.id_num = 1 self._generateUI() def _generateUI(self): main_widget = QWidget() self.main_layout = QVBoxLayout() main_widget.setLayout(self.main_layout) self.setCentralWidget(main_widget) button = QPushButton("button" + str(self.id_num)) self.main_layout.addWidget(button) add_button = QPushButton("+") add_button.clicked.connect(self._addItem) self.main_layout.addWidget(add_button) def _addItem(self): # put functions to insert widget here def launch(): app = QApplication.instance() if not app: app = QApplication(sys.argv) widget = MyMainWindow() widget.show() app.exec_() launch()
How to insert widget
Use insertWidget() to insert widget to the layout. This is an wxample of inserting at the second space from the top of the layout. If you’d like to insert widgets the top of the layout, the argument index should be 0.
def _addItem(self): self.id_num += 1 button = QPushButton("button" + str(self.id_num)) self.main_layout.insertWidget(1, button)
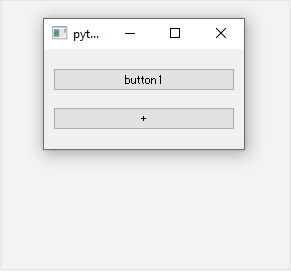
The first argument of insertWidget() “index” can’t be set from the end of the layout.
Inserts widget at position index, with stretch factor stretch and alignment alignment. If index is negative, the widget is added at the end.
from Qt Documentation
How to get index from the end
If you’d like to insert item at the n-th position from the end, you should set index (number of widgets – n)
Use count() to get the number of widget. So rewrite insertWidget() as below.
def _addItem(self): self.id_num += 1 button = QPushButton("button" + str(self.id_num)) self.main_layout.insertWidget(self.main_layout.count()-1, button)
The result is the following.
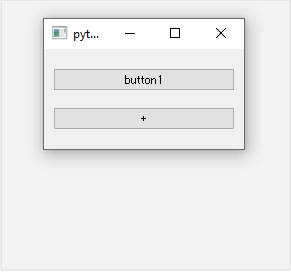