Create search.php for original theme
This is one of the articles about project “Create Original Theme in WordPress”.
GOAL
To create search.php and searchform.php
Environment
WordPress 5.5.1
XAMPP 7.4.10
search.php
It is easy to create search.php by copying and changing from archive.php.
<?php /** * The template to display search results *@package WordPress *@subpackage Techblog *@since Techblog 1.0 */ get_header(); ?> <div class="container"> <main id="main" class="site-main"> <div class= "main-content"> <?php if ( have_posts() ) :?> <h1><?php echo esc_html__( 'Search Results for: ', 'techblog' ).'<span>'.esc_html(get_search_query()).'</span>'; ?></h1> <?php while ( have_posts() ) : the_post(); get_template_part( 'content/content', 'search'); endwhile; the_posts_pagination( array('prev_text' => '<u>Back<<</u> ', 'next_text' => ' <u>>>Next</u>', ) ); endif; ?> </div> </main> <?php get_sidebar(); ?> </div> <?php get_footer(); ?>
Functions
get_search_query()
This function is used to get the posted search query from the search form. The definition is in wp-includes/general-template.php.
function get_search_query( $escaped = true ) { $query = apply_filters( 'get_search_query', get_query_var( 's' ) ); if ( $escaped ) { $query = esc_attr( $query ); } return $query; }
get_query_var( ‘s’ ) is the function to get the value of a query variable from the key ‘s’. So the input form should be named ‘s’.
get_template_part( $slug, $name = null, $args = array() )
This function is used to get template from {}-{}.php.
In my case, get the template from content/content-search.php by using get_template_part( ‘content/content’, ‘search’). But I don’t need content-search.php because the template content/content-search.php is the same as content.php.
Result
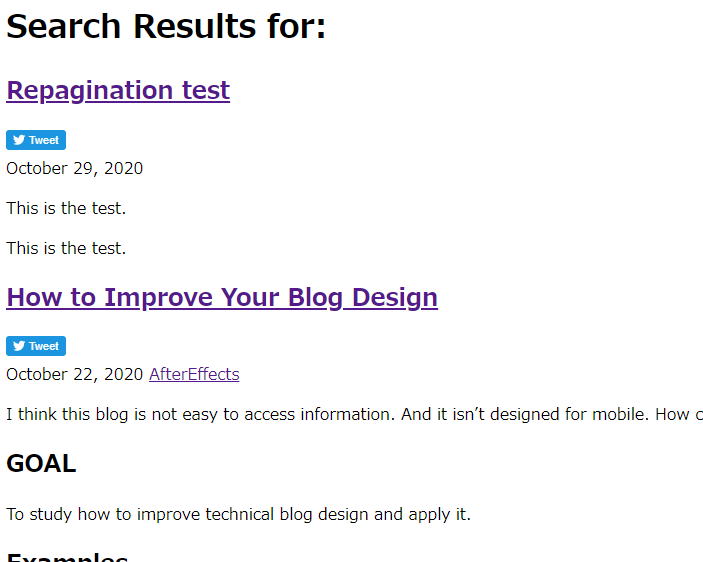
searchform.php
searchform is used as one of the widgets.
<?php /** * The search form template *@package WordPress *@subpackage Techblog *@since Techblog 1.0 */ ?> <form role="search" method="get" class="search-form" action="<?php echo esc_url( home_url( '/' ) ); ?>"> <div class="search-group"> <label class="screen-label" for="s"><?php esc_html_e( 'Search:', 'techblog' ); ?></label><br/> <span> <input type="text" class="search-query" placeholder="<?php esc_attr_e('word', 'techblog;' ); ?>" value="<?php echo get_search_query(); ?>" name="s" title="<?php esc_attr_e( 'Search for:', 'techblog' ); ?>"/> <button type="submit" class="search-submit" name="submit" value="<?php esc_attr_e( 'Search', 'techblog' ); ?>"><span class="material-icons">search</span></button> </span> </div> </form>
Result
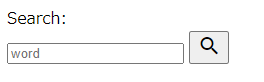
Change the style
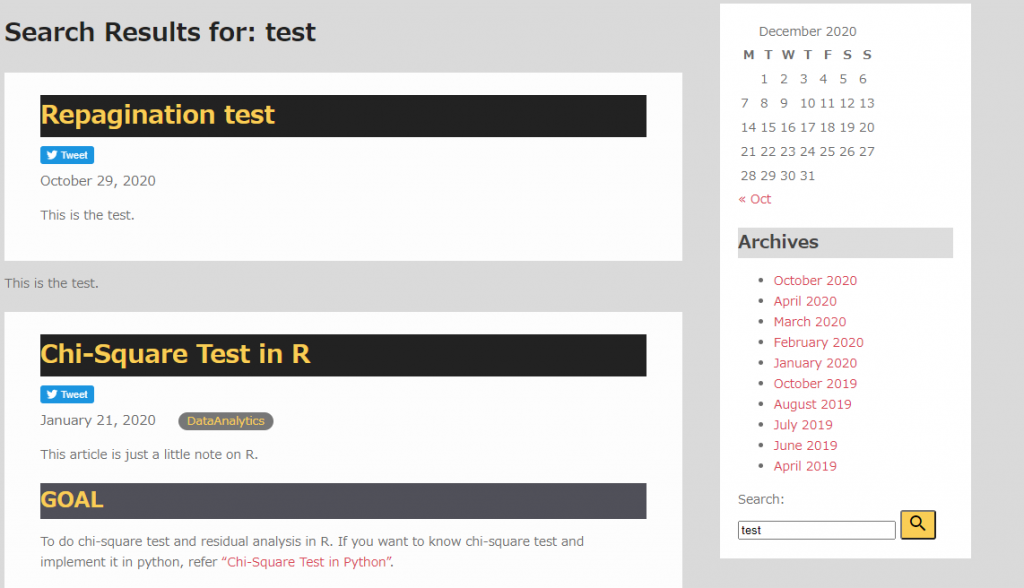