Create comments.php for original theme
This is one of the articles about project “Create Original Theme in WordPress”.
GOAL
To create comments.php
Environment
WordPress 5.5.1
XAMPP 7.4.10
Method
WordPress has useful function to display comment form and functions to post comments.
comments.php
<?php /** * The comment form for techblog. * @package WordPress * @subpackage Techblog * @since Techblog 1.0 */ if ( post_password_required() ) { return; }?> <div class="comment-area"> <div id="comments" class="comment-main"> <?php if ( have_comments() ) : ?> <h3 class="comments-title"> <?php echo "Comments for ".get_the_title(); ?> </h3> <ol class="comment-list"> <?php wp_list_comments( array( 'style' => 'ol', 'short_ping' => true, ) ); ?> </ol> <?php endif;?> <?php if (!comments_open() && get_comments_number() != '0' && post_type_supports( get_post_type(), 'comments' ) ) :?> <p class="no-comments"><?php esc_html_e( 'Comments are closed.', 'techblog' ); ?></p> <?php endif; comment_form(); ?> </div> </div>
Functions
post_password_required()
post_password_required() is used to protect contents (posts) with password. This function detect whether post requires password and correct password has been provided.
have_comments()
This returns true if comments are available for current WordPress query.
wp_list_comments($args = array(), $comments = null)
This function is used to displays a list of comments for current post or page. The argument array $args defines formatting options.
Reference: Parameters of wp_list_comments() in wordpress.org
comments_open()
This returns true if the current post is open for comments.
get_comments_number()
This function return string that represent the number of comments for the current post or page.
post_type_supports( $post_type, $feature )
This function checks a post type’s support for a given feature. The first argument $post_type is the post type being checked and the second one $feature is the feature being checked.
In my case, get the post type of the current post with get_post_type(), and check if it is ‘comments’.
comment_form( $args = array(), $post_id = null )
comment_form() is used to output a complete commenting form for use within a template.
This function is used to displays a list of comments for current post or page. The argument array $args defines default arguments and form fields to override. You can use it to change class and id names.
Reference: Parameters of comment_form() in wordpress.org
Result
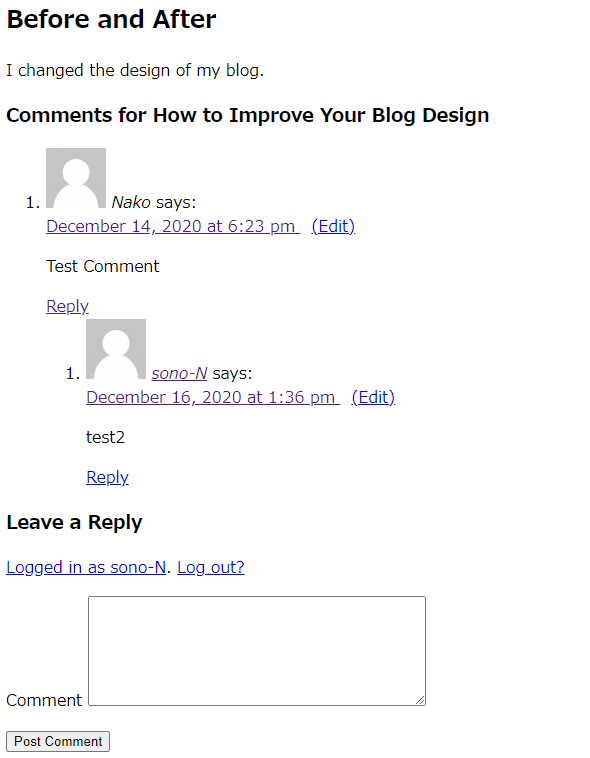
Change the style
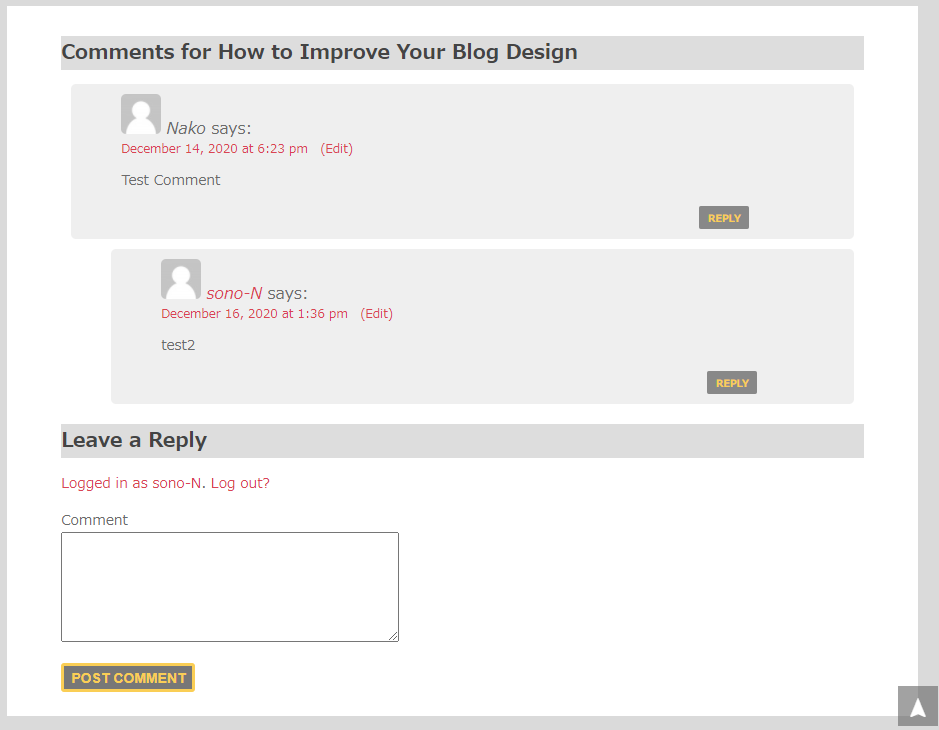
Hey very interesting blog!