What is Cross-Origin Resource Sharing (CORS)
GOAL
To understand Cross-Origin Resource Sharing (CORS) and how to avoid error regarding it.
Reference
Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing (CORS)
Cross-Origin Resource Sharing is sharing resources from a different origin by using additional HTTP headers to allow a web application to access to that resources.
What is origin?
Origin is the currency to decide resource sharing and isolating.
Usually actors in the Web platform that share the same origin trust each other and have the same authority.
Origin is defined by the scheme, host and port of the URL of the resource. Scheme is client server protocol, http or https.
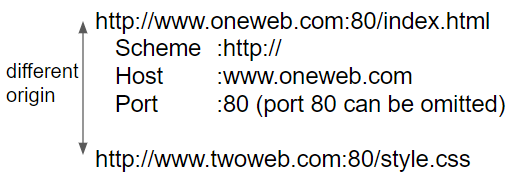
They are examples of same-origin and different-origin.
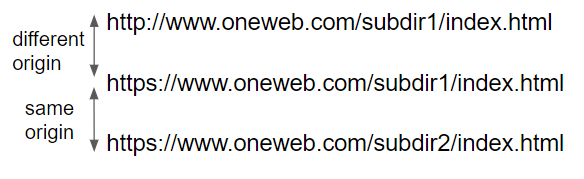
What is origin for?
When a browser need to get resource from a server, the browser should check whether the server is different from the one where index.html is. That’s because access to the cross-origin server can causes cross-site printing. The rule is called cross-origin policy.
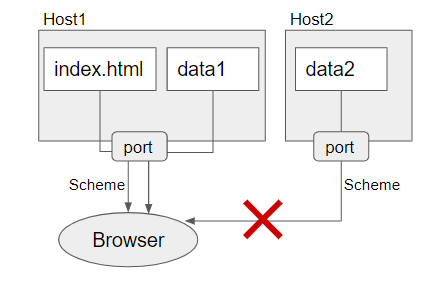
Mechanism of CORS
Client (Web browser) adds “Origin” to HTTP Request header. If the origin is included in Access-Control-Allow-Origin of HTTP Response header, data can be transmitted.
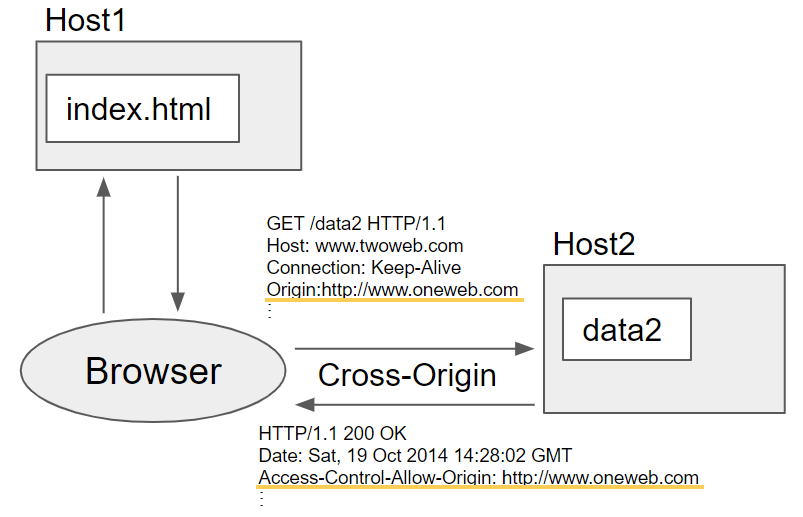
You can see more about HTTP request/response more in “What is http request/response.”
Preflighted requests
There are 3 types of Cross-Origin Request.
1 Simple requests
In cross-origin resource sharing, browser uses the HTTP OPTION request method to preflight the request. Preflighting is to check if a request is accepted to the server before sending it.
However, simple requests that meet some conditions don’t trigger preflight. There are multiple conditions, and you can see them from the link below.
“Simple requests” from https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS
2 Preflighted requests
If the request from browser is not simple request, browser first send an HTTP request by the OPTIONS method. Then server responses to preflight request. After preflight request is complete, the real request is sent:
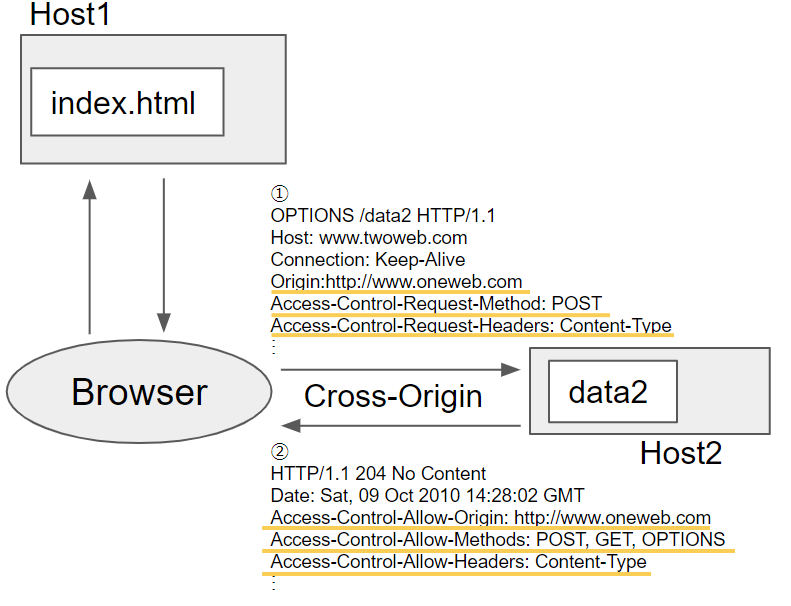
The value of Origin in request should be contained in Access-Control-Allow-Origin in response.
The value of Access-Control-Request-Method should be contained in Access-Control-Allow-Methods in response
The values of Access-Control-Request-Headers should be contained in Access-Control-Allow-Headers in response.
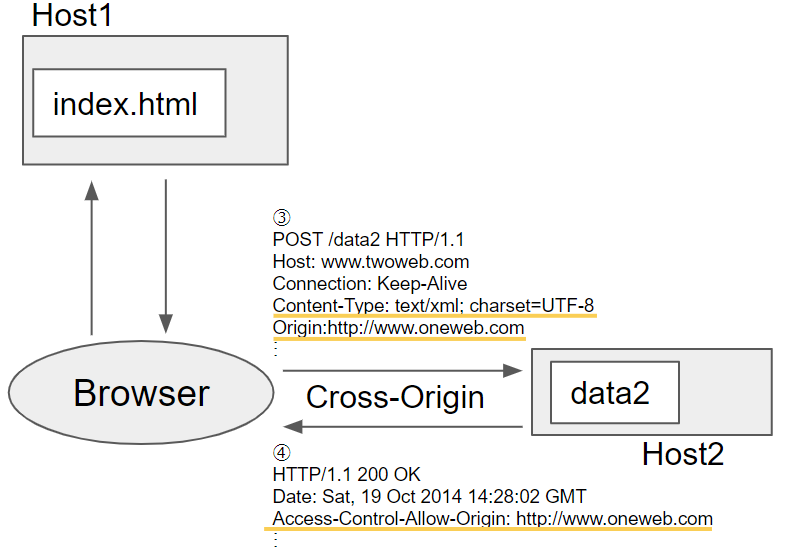
3 Requests with credentials
Browser can send request that are aware of HTTP cookies and HTTP Authentication information.
If web application needs a resource on cross-origin server which sets Cookies, browser send GET request with Origin and Cookie header. Then server send response with Access-Control-Allow-Origin, Access-Control-Allow-Credentials(true) and Set-Cookie header.
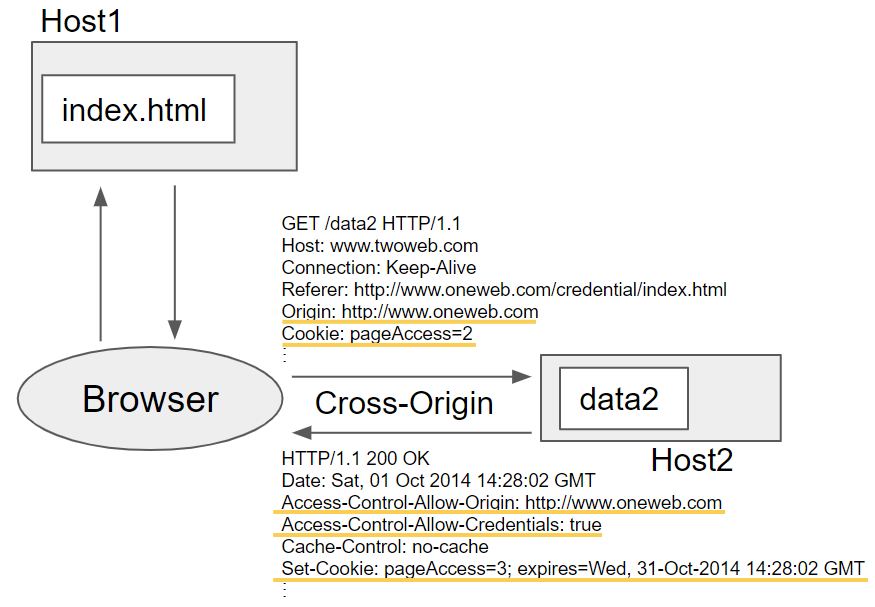
The origin of Access-Control-Allow-Origin should be the same one as the value of Origin in the request header.
Problems with CORS
You can see some errors and solutions in “CORS errors” from Web technology for developers document.
Problem with WebGL texturing
In my case, the error below occurred in WebGL texture call.
Uncaught DOMException: Failed to execute 'texImage2D' on 'WebGLRenderingContext': The image element contains cross-origin data, and may not be loaded.
How to solve this problem
If it works on the server, you should add crossOrigin to image.
image.src = url; image.crossOrigin = origin; #origin is the domain where the image exists
If it works on local development environment (directory on your HDD), you should set up a server.
Use php or python command
Open terminal and input command.
1. python
cd <path to the directory where index.html and resource exists> python -m SimpleHTTPServer 8080
2. php
cd <path to the directory where index.html and resource exists> php -S localhost:8080
Use XAMPP
XAMPP is a free and open-source cross-platform web server solution package.