Memorandum of Shadertoy Learning. part1
I’m trying shadertoy these days. This is my tutorial for shadertoy.
1 main function
void mainImage( out vec4 fragColor, in vec2 fragCoord ) is the entry point in Shadertoy.
void mainImage( out vec4 fragColor, in vec2 fragCoord ) { //main drawing process is here }
Variable fragCoord contains the pixel coordinates for which the shader needs to compute a color. The resulting color is gathered in fragColor as a four component vector
Modifiers
- in
- an “in” variable is used only in this function and not output.
- out
- an “out” variable is an output variable that can be passed by reference.
- inout
- an “inout” variable is an output and input variable.
2 fragColor
void mainImage( out vec4 fragColor, in vec2 fragCoord )
{
fragColor = vec4(0.98, 0.808, 0.333, 1.0);
}
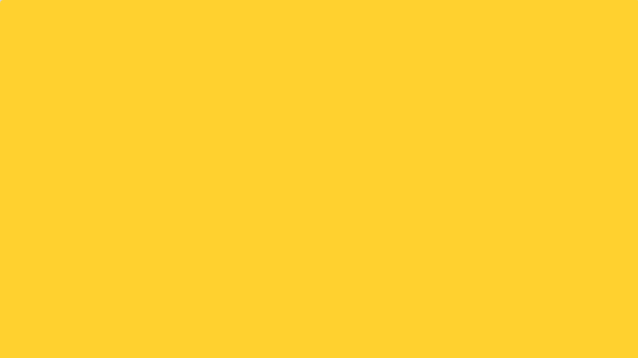
Assign vec4 (R, G, B, alpha) to fragColor variable.
3 fragCoord
variable fragCoord is coordinate of target pixel. fragCoord.x is x coordinate and fragCoord.y is y coordinate and fragCoord.xy is (x,y) coordinate.
void mainImage( out vec4 fragColor, in vec2 fragCoord ) { vec3 color1 = vec3(1.0, 1.0, 0.5); vec3 color2 = vec3(0.98, 0.808, 0.333); if (int(fragCoord.x )% 50 < 25){ fragColor = vec4(color1, 1.0); } else{ fragColor = vec4(color2, 1.0); } }
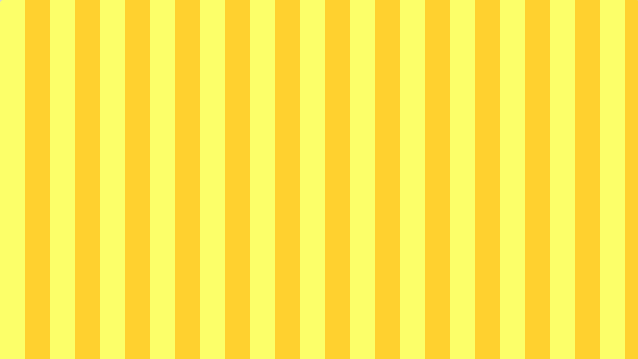
4 iResolution
Uniform variable iResolution passes the resolution of the image to the shader. iResolution.x is x coordinate and iResolution.y is y coordinate and iResolution.xy is (x,y) coordinate.
void mainImage( out vec4 fragColor, in vec2 fragCoord ) { int n = 4; int width = int(iResolution.x)/n; if (int(fragCoord.x )% width < width/2){ fragColor = vec4(1.0, 1.0, 1.0-fragCoord.y/iResolution.y, 1.0); } else{ fragColor = vec4(1.0, 0.0, fragCoord.y/iResolution.y, 1.0); } }
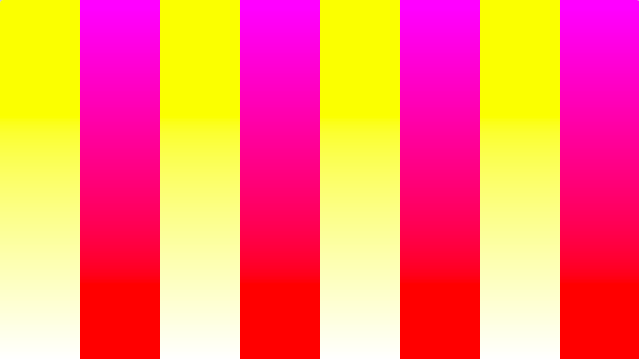
5 Conditional branch and color setting
Check the article “How to Convert HSV to RGB” for more information about hsv2rgb.
vec3 hsv2rgb(in vec3 hsv){ vec3 rgb; float h = hsv.x * 360.0; float s = hsv.y; float v = hsv.z; float c =v * s; float h2 = h/60.0; float x = c*(1.0 - abs( mod(h2, 2.0) - 1.0)); switch(int(h2)){ case 0: rgb = vec3(v-c) + vec3(c, x, 0.0); return rgb; case 1: rgb = vec3(v-c) + vec3(x, c, 0.0); return rgb; case 2: rgb = vec3(v-c) + vec3(0.0, c, x); return rgb; case 3: rgb = vec3(v-c) + vec3(0.0, x, c); return rgb; case 4: rgb = vec3(v-c) + vec3(x, 0.0, c); return rgb; case 5: rgb = vec3(v-c) + vec3(c, 0.0, x); return rgb; case 6: rgb = vec3(v-c) + vec3(c, x, 0.0); return rgb; } } void mainImage( out vec4 fragColor, in vec2 fragCoord ) { vec2 coordinate = fragCoord.xy - 0.5*iResolution.xy; vec3 pixel_color = vec3(0.5); int gradation = 10; int r_width = int(0.5*iResolution.y/float(gradation)); int r; for(int i =0; i<gradation+1; i++){ r = r_width*i; float i_flaot = float(float(i)/float(gradation+1)); if(coordinate.x*coordinate.x + coordinate.y*coordinate.y < float(r*r)){ pixel_color = hsv2rgb(vec3((1.0/float(gradation))*float(i), 1.0, 1.0)); break; } } fragColor = vec4(pixel_color, 1.0); }
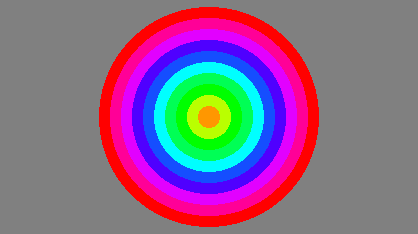